Tuesday, January 28, 2025
Valid Anagram
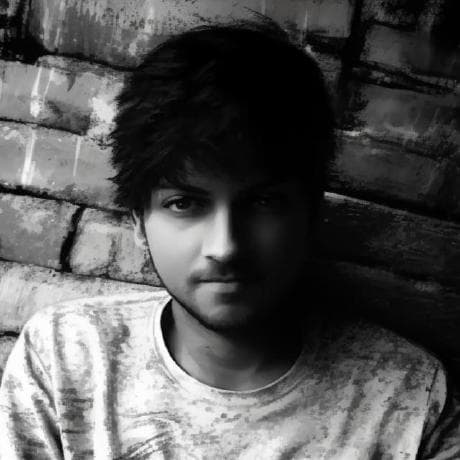
Updated: January 2025
Valid Anagram
easy
💡 Intuition
- By sorting both strings, we can compare their characters at each index. If both strings are valid anagrams, their sorted forms will match. This approach has a time complexity of O(n log n) for sorting and (O(n)) for comparison, resulting in an overall complexity of O(n log n).
- Alternatively, we can count the occurrences of each character in the first string and compare it with the character counts in the second string. This involves one loop to count characters and another loop to validate the counts, giving a time complexity of ( O(n + n) = O(n) ).
🚀 Solution
go
⏳ Time Complexity
- Since we are taking single loop for the array of length n, the time complexity will be
O(n)